· programming, telegram · 7 min read
Developing a simple Telegram mini app
Experiment summary
I’ve experimented with creating a simple Telegram mini-app. My goal was to understand how they work and whether the Telegram platform is a good choice for investing time in developing such apps.
To save you time, before diving into the details of the implementation and explaining what a Telegram mini-app is, here’s a quick summary of my experiment:
It’s not worth investing time in developing such apps if your primary goal is gaining additional (or any) visibility for your app. If you’re looking to expand your audience by leveraging the Telegram app store, think twice—it may not be worth the effort. The review process for getting listed takes at least three months, which is a significant delay for many developers.
Mini-apps, however, can complement regular Telegram bots, especially if they bring additional value through a custom UI or require Telegram-specific features like in-app payments. They shine when you need more sophisticated functionality than what standard bots can provide.
In simple terms, a mini-app is any web app or web page. All you need is a link to your app or page. For example, if you’ve built a React app, you can deploy it to a service like Cloudflare Pages and then provide the app’s link to Telegram via BotFather. But if your goal is to get listed in the Telegram mini-app store, things get more complex—you’ll need to meet their detailed requirements, which can be time-consuming.
Understanding Telegram Mini-Apps
As mentioned earlier, Telegram Mini-Apps are essentially add-ons for Telegram Bots, designed to enhance user interaction. To put it simply, while bots handle the logic and functionality—like telling jokes, generating random numbers, or helping users buy movie tickets—Mini-Apps take care of the visual and interactive part.
The challenge with standard bots lies in their interface. They operate much like a command-line tool, which works well for developers but can feel clunky and unintuitive for everyday users. This is where Mini-Apps step in.
By leveraging Mini-Apps, developers can create sleek, user-friendly, and interactive interfaces that feel modern and intuitive. Instead of relying on plain text commands and simple button lists, Mini-Apps open up possibilities for visually rich designs and dynamic functionality. Think of them as tiny web apps embedded directly into Telegram—flexible and functional.
When to Use a Mini-App?
A Mini-App is particularly useful when a bot’s standard interface isn’t enough. If the user experience demands something more interactive, like multi-step forms, dynamic data visualization, or a shopping experience with an integrated payment system, a Mini-App is the way to go. It’s the tool of choice when simplicity won’t cut it, and you need to offer functionality beyond just displaying buttons or sending plain messages.
What Makes Mini-Apps Powerful?
Mini-Apps can leverage modern web technologies like JavaScript to build advanced interfaces that load directly inside Telegram. What makes this even more compelling is the integration with Telegram’s ecosystem. Mini-Apps come with a host of built-in features:
Seamless Authorization: Users can log in to your Mini-App with their Telegram credentials, eliminating friction and simplifying the user experience.
Integrated Payments: With support for 20 payment providers, including Google Pay and Apple Pay, Mini-Apps make transactions smooth and straightforward. This is particularly valuable for e-commerce, subscriptions, or any monetized service.
Push Notifications: Tailored notifications keep users engaged, whether it’s updates, reminders, or promotional offers.
Viral and Retention Mechanics: By being embedded within Telegram, Mini-Apps can tap into Telegram’s social and viral potential, enabling direct advertising and user acquisition mechanics right inside the app.
Crypto Support: For businesses dealing with cryptocurrencies, Mini-Apps support integrated crypto payments, providing flexibility for global transactions.
In essence, a Telegram Mini-App can function as a complete replacement for a website or standalone app, allowing businesses to engage with Telegram’s vast user base without ever leaving the messenger. This seamless integration can be a game-changer for brands looking to embed themselves into users’ daily lives.
My mini-app implementation
I created a single page using React and a framework specifically designed for mini-apps. As I mentioned earlier, a mini-app is essentially just a link to your web app or page. It can be built with anything—React, Next.js, Astro, Rust, plain HTML, you name it.
When starting out, I had two main technology choices:
- React, or
- A mini-apps framework.
I decided to go with the framework because it offered additional features that made development more straightforward. What makes this platform interesting is the wide range of tools it offers to integrate your web app seamlessly into Telegram. These tools allow you to make a Mini App feel like a native part of the Telegram interface, simulating the behavior and appearance of a native app.
In other words, the framework offers UI elements that resemble native Telegram components and takes care of cross-platform compatibility for you.
Idea
For this experiment, I wanted to keep things simple. I came up with the idea of a single page that tracks the time a user spends on it—just focusing on their thoughts, feelings, or simply doing nothing. The page itself doesn’t do much beyond counting time and displaying a few blocks of text.
Here’s the page’s code:
import { FC, useEffect, useState } from 'react';
import { retrieveLaunchParams } from '@telegram-apps/sdk';
import { Page } from '@/components/Page';
import './IndexPage.css';
export const IndexPage: FC = () => {
const [timeSpent, setTimeSpent] = useState(0);
const [message, setMessage] = useState('');
const randomMessages = [
'Maybe think about that thing.',
'Enjoy.',
'Take a deep breath.',
'Consider your next move.',
];
useEffect(() => {
const timeInterval = setInterval(() => {
setTimeSpent((prev) => prev + 1);
}, 1000);
return () => clearInterval(timeInterval);
}, []);
useEffect(() => {
const changeMessage = () => {
const randomIndex = Math.floor(Math.random() * randomMessages.length);
setMessage(randomMessages[randomIndex]);
};
changeMessage();
const messageInterval = setInterval(changeMessage, 60000);
return () => clearInterval(messageInterval);
}, []);
useEffect(() => {
const { initData } = retrieveLaunchParams();
if (initData?.user) {
const u = initData.user;
fetch('https://my-cloudflare-worker.com/saveUser', {
method: 'post',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
firstName: u.firstName,
lastName: u.lastName,
username: u.username,
photoUrl: u.photoUrl,
languageCode: u.languageCode,
}),
}).catch(e => console.error(e));
}
}, []);
const formatTime = (seconds: number): string => {
const hours = Math.floor(seconds / 3600);
const minutes = Math.floor((seconds % 3600) / 60);
const remainingSeconds = seconds % 60;
if (hours) return `${hours} hours${hours > 1 ? 's' : ''} ${minutes} minutes`;
if (minutes) return `${minutes} minutes ${remainingSeconds} seconds`;
return `${remainingSeconds} seconds`;
};
return (
<Page back={false}>
<div className="index-page">
<div className="index-page__content">
<p className="index-page__text">You've been present with yourself for:</p>
<p className="index-page__counter">{formatTime(timeSpent)}</p>
<p className="index-page__message">{message}</p>
</div>
</div>
</Page>
);
};
The page displays a counter, updates random text every minute, and records the Telegram user visiting the mini-app. It connects to a Cloudflare worker, which saves user information in a D1 SQL database. I’m storing basic user data to track page usage, as Telegram doesn’t offer this functionality out of the box.
The result looks like this:
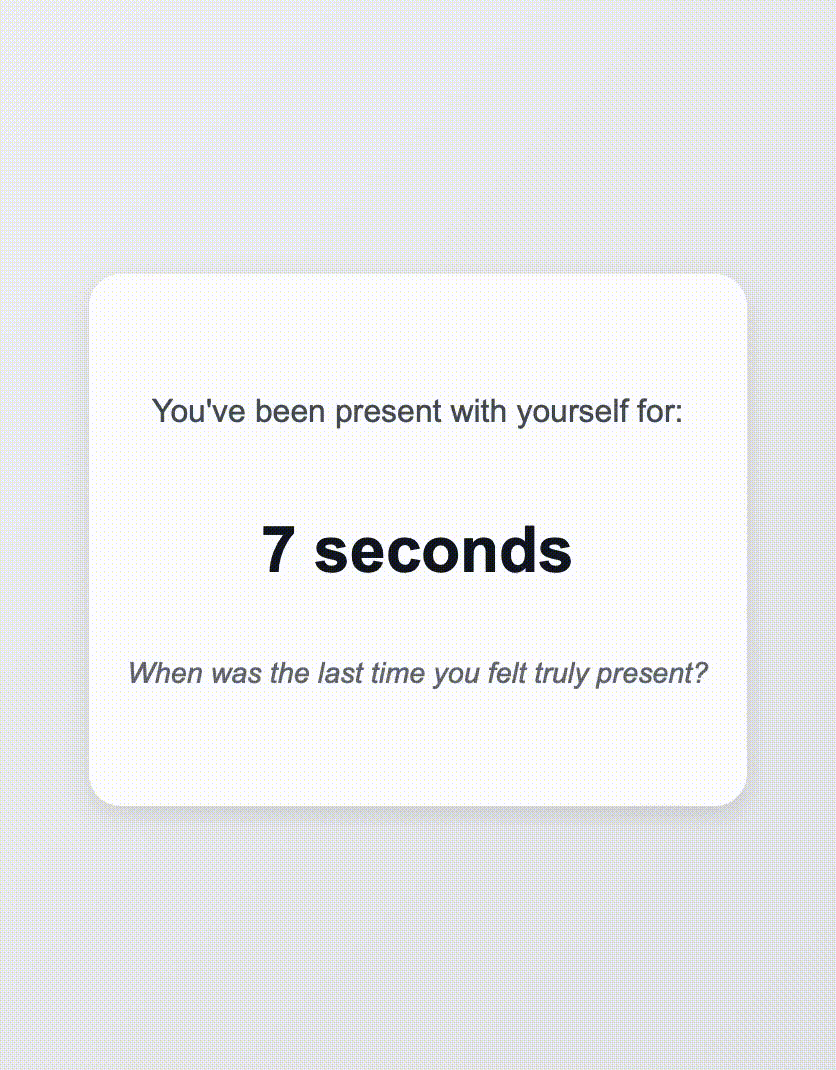
Telegram Apps Center
This is essentially Telegram’s version of an “app store.” Here’s what you should know if you’re considering leveraging it. Telegram’s goal with the Apps Center is to establish its own platform for (mini) apps, taking advantage of the messenger’s massive user base of millions of active users. According to Telegram, the main benefit of creating a mini-app is the potential to “unlock access to 900 million Telegram audience”.
The idea is that the Apps Center provides developers with a platform to reach this audience. However, there are some critical questions to consider:
With a review time of at least three months and an unclear review process, how can developers depend on this platform? After all, it’s not a typical mobile app; it’s a highly constrained solution that works only within the Telegram messenger.
The Apps Center appears to focus mostly on crypto-related mini-apps (Web3, Play-to-Earn categories). It’s unclear if there’s much interest in “regular” solutions.
Summary
Since I don’t have an existing bot to build a mini-app for, this experiment was more about understanding the platform’s potential. Unless the app store improves its review times to deliver real value—like increased visibility —- it’s hard to justify investing time in creating mini-apps.
That said, if you already have a useful bot, adding a mini-app to extend its functionality and provide additional value can be a logical and worthwhile step.